Components on Blazor
Hi my fellow Padawans
Blazor is a frontend framework like Angular or React but mostly like React and it has components for create pages and reusable objects functionalities.
But how can we create a component and use it on the pages in our Blazor application?
First Read this Article and create your first Blazor application 🙂
Then on your Solution Explorer find Pages and right click on it and Add -> Razor Component
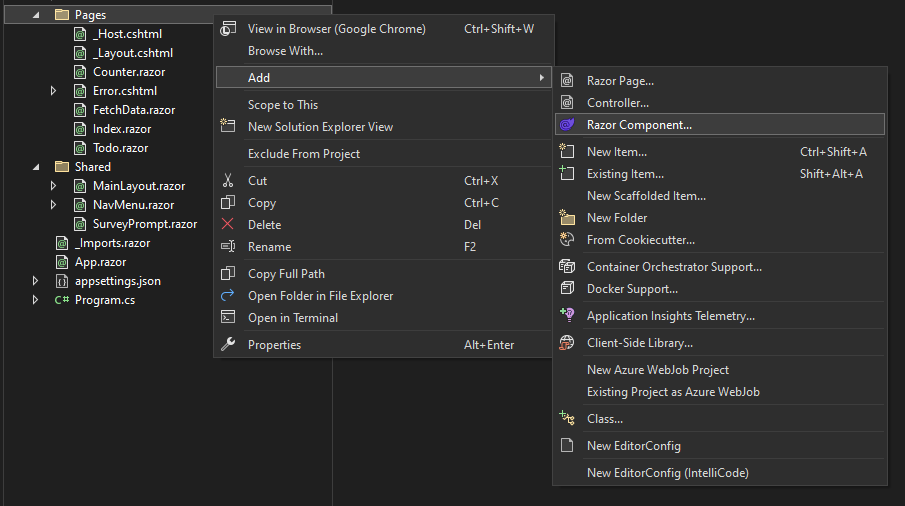
On Add New Item windows write your components name and create it
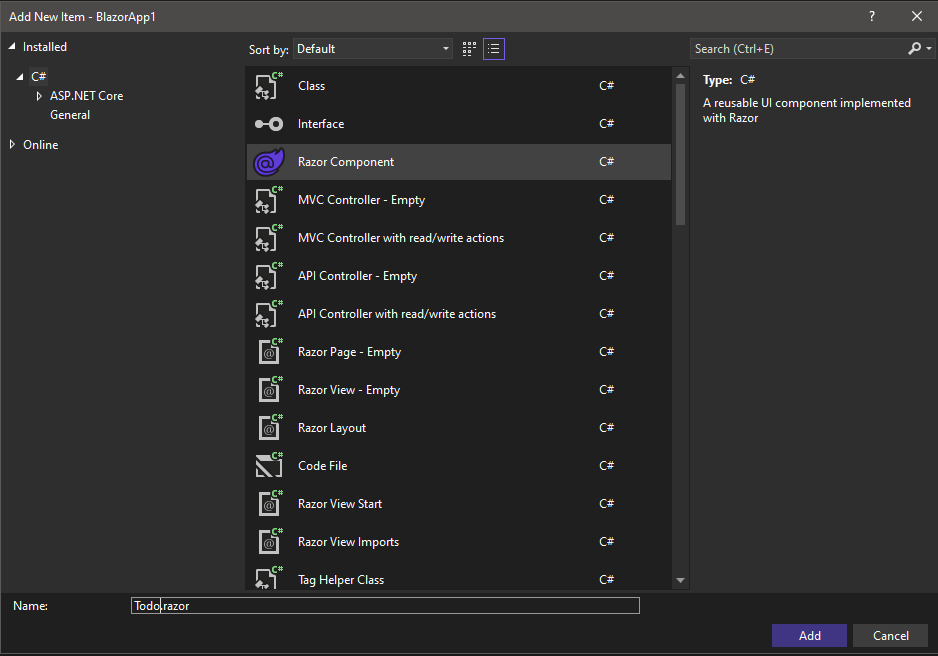
I decided to make something different and I created Todo.Razor file and I add these codes on the page
@page "/todo"
@using BlazorApp1.Data;
<h3>Todo</h3>
<ul>
@foreach (var todo in todos)
{
<li>@todo.Title</li>
}
</ul>
<input placeholder="Something todo" @bind="newTodo" />
<button @onclick="AddTodo">Add todo</button>
@code {
private List<TodoItem> todos = new();
private string? newTodo;
private void AddTodo()
{
if (!string.IsNullOrWhiteSpace(newTodo))
{
todos.Add(new TodoItem { Title = newTodo });
newTodo = string.Empty;
}
}
}
And find NavMenu.razor file inside of the Shared folder on your Solution Explorer. Find the last item of menu divs and Add this code:
<div class="nav-item px-3">
<NavLink class="nav-link" href="todo">
<span class="oi oi-list-rich" aria-hidden="true"></span> Todo
</NavLink>
</div>
You will have a NavMenu.Razor page like this:
<div class="top-row ps-3 navbar navbar-dark">
<div class="container-fluid">
<a class="navbar-brand" href="">BlazorApp1</a>
<button title="Navigation menu" class="navbar-toggler" @onclick="ToggleNavMenu">
<span class="navbar-toggler-icon"></span>
</button>
</div>
</div>
<div class="@NavMenuCssClass" @onclick="ToggleNavMenu">
<nav class="flex-column">
<div class="nav-item px-3">
<NavLink class="nav-link" href="" Match="NavLinkMatch.All">
<span class="oi oi-home" aria-hidden="true"></span> Home
</NavLink>
</div>
<div class="nav-item px-3">
<NavLink class="nav-link" href="counter">
<span class="oi oi-plus" aria-hidden="true"></span> Counter
</NavLink>
</div>
<div class="nav-item px-3">
<NavLink class="nav-link" href="fetchdata">
<span class="oi oi-list-rich" aria-hidden="true"></span> Fetch data
</NavLink>
</div>
<div class="nav-item px-3">
<NavLink class="nav-link" href="todo">
<span class="oi oi-list-rich" aria-hidden="true"></span> Todo
</NavLink>
</div>
</nav>
</div>
@code {
private bool collapseNavMenu = true;
private string? NavMenuCssClass => collapseNavMenu ? "collapse" : null;
private void ToggleNavMenu()
{
collapseNavMenu = !collapseNavMenu;
}
}
When you open the webpage you will see the Todo on your left navigation menu and then you will see your amazing Todo application like below:
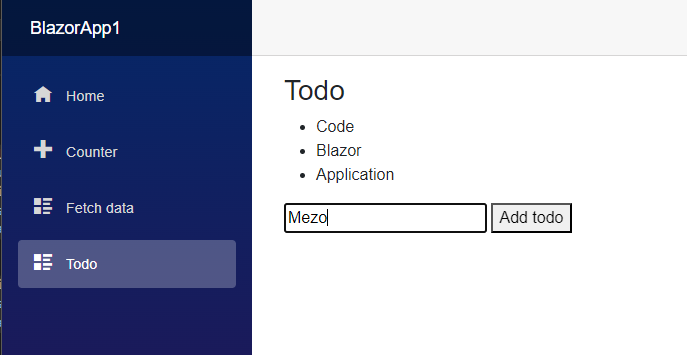
If you want to use this component in other pages for example add this component on the home page. Go to your Index.Razor inside of the Pages folder and add this code :
<Todo/>
Your index.Razor will look like this
@page "/"
<PageTitle>Index</PageTitle>
<h1>Hello, Everyone!</h1>
Welcome to your new app. Hello
<SurveyPrompt Title="How is Blazor working for you?" />
<Todo/>
Then we RUN our app oooorrr we can Hot Reload and we will see the result like below:

That is easy isn’t it ?
I hope that is helpful
May the knowledge be with you